Plugin Development Introduction¶
この章ではプラグインの設計と書き方の基礎について説明します。この章を読むことは下記の理解の手助けになるでしょう。
非同期イベントモード。 Traffic Server 全体で用いられる設計パラダイムです。プラグインもこの設計に従っていなければなりません。これは Traffic Server がプラグインを "起こして" 処理を実行するコールバックメカニズムを含みます。
HTTP ステートマシンの概要を伴う Traffic Server の HTTP 処理
プラグインがフックを入れて、 Traffic Server の HTTP 処理を変更 / 拡張する方法
Traffic Server API により提供される機能の概要を伴う Roadmap
Roadmap¶
This chapter has provided an overview of Traffic Server's HTTP processing, API hooks, and the asynchronous event model. Next, you must understand the capabilities of Traffic Server API functions. These are quite broad:
HTTP header manipulation functions
Obtain information about and manipulate HTTP headers, URLs, & MIME headers.
HTTP transaction functions
Get information about and modify HTTP transactions (for example: get the client IP associated to the transaction; get the server IP; get parent proxy information)
IO functions
Manipulate vconnections (virtual connections, used for network and disk I/O)
Network connection functions
Open connections to remote servers.
Statistics functions
Define and compute statistics for your plugin's activity.
Traffic Server management functions
Obtain values for Traffic Server configuration and statistics variables.
Below are some guidelines for creating a plugin:
Decide what you want your plugin to do, based on the capabilities of the API and Traffic Server. The two kinds of example plugins provided with this SDK are HTTP-based (includes header-based and response transform plugins), and non-HTTP-based (a protocol plugin). These examples are discussed in the following chapters.
Determine where your plugin needs to hook on to Traffic Server's HTTP processing (view the HTTP トランザクションの状態遷移図).
Read Header-Based Plugin Examples to learn the basics of writing plugins: creating continuations and setting up hooks. If you want to write a plugin that transforms data, then read HTTP Transformations.
Figure out what parts of the Traffic Server API you need to use and then read about the details of those APIs in this manual's reference chapters.
Compile and load your plugin (see はじめに).
Depending on your plugin's functionality, you might start testing it by issuing requests by hand and checking for the desired behavior in Traffic Server log files. See the *Traffic Server Administrator's Guide* for information about Traffic Server logs.
Asynchronous Event Model¶
Traffic Server はマルチスレッドで動作するプロセスです。サーバがマルチスレッドを使用する主な理由は二つあります。
複数 CPU と複数 I/O デバイスが使用可能な平行性の利点を得るため
同時に大量のクライアントコネクションを持つことによる平行性を管理するため。例として、OS にスレッドの切り替えを制御させることでコネクション毎に一つのスレッドを作成できます。
Traffic Server は最初の理由の為に複数スレッドを使用します。しかしながら数千の同時コネクションを処理する際に効率が悪くなるため、 Traffic Server はトランザクション処理毎の OS スレッドの分離を行いません。
代わりに、効率的な処理のスケジューリングのために Traffic Server は特殊なイベント駆動メカニズム、イベントシステムと継続を提供します。 イベントシステム はスレッド上で行われる処理のスケジューリングに使用されます。 継続 は待機点に到達するまで幾つかの処理を実行可能な、受動的なイベント駆動ステートマシンです。これは更なる処理を行うのに適した状態の通知を受け取るまでスリープします。例として、 HTTP ステートマシン( HTTP トランザクションを処理するもの)は継続として実装されます。
継続オブジェクトは Traffic Server 全体で使用されます。その幾つかは Traffic Server プロセスが実行中ずっと生存する可能性がありますが、他のものは特定の要求に合わせて(おそらくは他の継続によって)生成され、その後破棄されます。 Traffic Server 内部構造 (下記)は Traffic Server の主要なコンポーネントがどのように影響し合うのかを示しています。 Traffic Server は、キャッシュやネットワーク I/O タスクを統合する キャッシュプロセッサー と ネットプロセッサー のような幾つかの プロセッサー を持ちます。プロセッサーはイベントシステムと対話し、スレッド上の作業をスケジュールします。実行スレッドは継続にイベントを送信することで継続をコールバックします。継続がイベントを受信した際、起動し、幾つかの処理を行い、自身を破棄するか再び眠り次のイベントを待ちます。
Traffic Server の内部構造
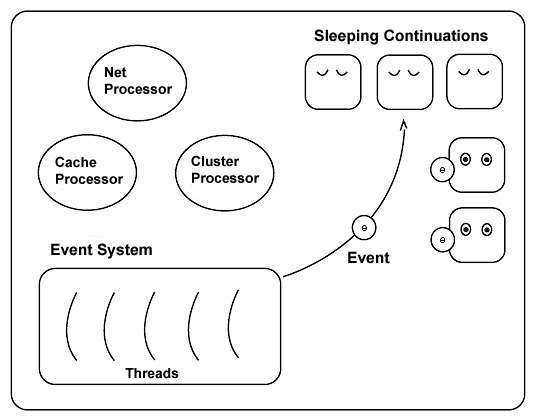
Traffic Server 内部構造¶
Plugins are typically implemented as continuations. All of the sample
code plugins (except hello_world
) are continuations that are created
when Traffic Server starts up; they then wait for events that trigger
them into activity.
プラグインと Traffic Server
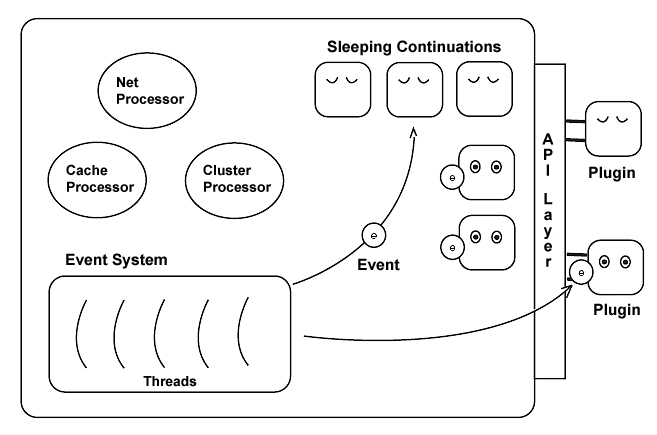
プラグインと Traffic Server¶
A plugin may consist of just one static continuation that is called
whenever certain events happen. Examples of such plugins include
denylist_1.cc
, basic_auth.cc
, and redirect_1.cc
.
Alternatively, a plugin might dynamically create other continuations as
needed. Transform plugins are built in this manner: a static parent
continuation checks all transactions to see if any are transformable;
when a transaction is transformable, the static continuation creates a
type of continuation called a vconnection. The vconnection lives as
long as it takes to complete the transform and then destroys itself.
This design can be seen in all of the sample transform plugins. Plugins
that support new protocols also have this architecture: a static
continuation listens for incoming client connections and then creates
transaction state machines to handle each protocol transaction.
プラグインを書く際、継続にイベントを送信する方法は幾つか存在します。 HTTP プラグインについては、 HTTP ステートマシンが必要に応じてプラグインを起こす呼び出しを送信することを可能にする "フック" のメカニズムが存在します。加えて、幾つかの Traffic Server API 関数、 TSContCall
、 TSVConnRead
、 TSCacheWrite
そして TSMgmtUpdateRegister
は Traffic Server サブプロセスがプラグインにイベントを送信するきっかけとなります。
Naming Conventions¶
Traffic Server HTTP ステートマシン¶
Traffic Server は高度な HTTP キャッシュとプロキシを行います。重要な特徴としてオルタネイトとドキュメントのフレッシュネスのチェック、フィルタリング、階層的キャッシュのサポート、そしてホスティングを含みます。 Traffic Server は同時に数千のクライアントリクエストを処理し、各リクエストは HTTP ステートマシンにより処理されます。これらのマシンは Traffic Server の機能をサポートするために要求される状態の全てを含む、複雑な状態遷移図に従います。 Traffic Server API は、プラグインとの関連を選択されたこれらの状態のサブセットへのフックを提供します。 HTTP トランザクションの状態遷移図 でAPI フックと関係する HTTP 状態が見られます。
この節(の下)の例ではプラグインの典型的な介入と Traffic Server の HTTP トランザクション処理の拡張方法について説明します。 Traffic Server の処理へのフックに関する完全な詳細は Hooks and Transactions にあります。
HTTP トランザクション¶
HTTP トランザクションはウェブドキュメントに対するクライアントリクエストと Traffic Server のレスポンスから成立します。レスポンスは要求されたウェブサーバーのコンテンツになるかも知れないし、エラーメッセージになるかも知れません。コンテンツは Traffic Server のキャッシュから配信されるかも知れませんし Traffic Server がオリジンサーバーから取得するであろうものから配信されるかも知れません。以下の図は典型的なトランザクション、特にコンテンツがキャッシュから配信される際のシナリオにおける幾つかの状態を示したものです。
簡略化された HTTP トランザクション
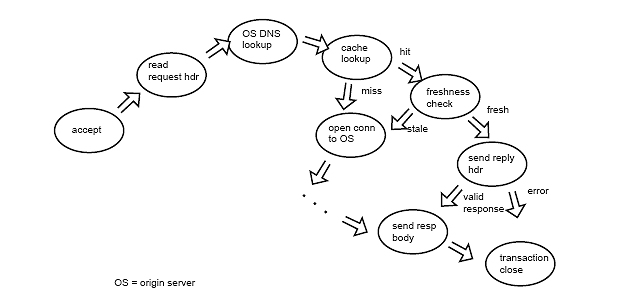
簡略化された HTTP トランザクション¶
上の図において、 Traffic Server はクライアントコネクションを accept し、リクエストヘッダーを読込み、オリジンサーバの IP アドレスをルックアップし、キャッシュ上でリクエストされたコンテンツを検索します。キャッシュにコンテンツがない場合( "ミス" )、 Traffic Server はオリジンサーバへのコネクションを open し、コンテンツのリクエストを発行します。キャッシュにコンテンツがある場合( "ヒット" )、 Traffic Server はフレッシュネスをチェックします。
If the content is fresh, then Traffic Server sends a reply header to the client. If the content is stale, then Traffic Server opens a connection to the origin server and requests the content. The figure above, 簡略化された HTTP トランザクション, does not show behavior in the event of an error. If there is an error at a any stage, then the HTTP state machine jumps to the "send reply header" state and sends a reply. If the reply is an error, then the transaction closes. If the reply is not an error, then Traffic Server first sends the response content before it closes the transaction.
API フックと状態の関係
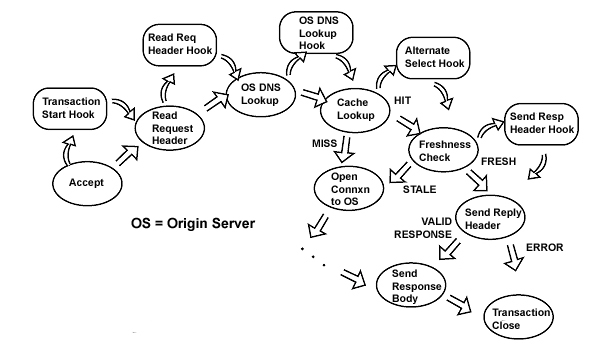
リストの状態に対応した API フック¶
You use hooks as triggers to start your plugin. The name of a hook reflects the Traffic Server state that was just completed. For example, the "OS DNS lookup" hook wakes up a plugin right after the origin server DNS lookup. For a plugin that requires the IP address of the requested origin server, this hook is the right one to use. The Denylist plugin works in this manner, as shown in the Denylist Plugin diagram below.
Denylist Plugin
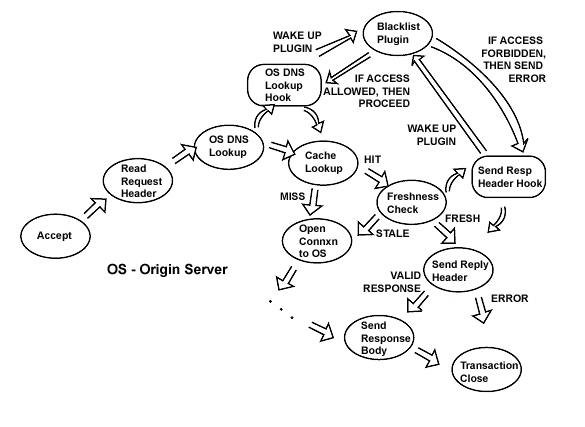
Denylist Plugin¶
Traffic Server calls the Denylist plugin right after the origin server DNS lookup. The plugin checks the requested host against a list of denylisted servers; if the request is allowed, then the transaction proceeds. If the host is forbidden, then the Denylist plugin sends the transaction into an error state. When the HTTP state machine gets to the "send reply header" state, it then calls the Denylist plugin to provide the error message that's sent to the client.
フックのタイプ¶
The Denylist plugin's hook to the origin server DNS lookup state is a global hook, meaning that the plugin is called every time there's an HTTP transaction with a DNS lookup event. The plugin's hook to the send reply header state is a transaction hook, meaning that this hook is only invoked for specified transactions (in the Denylist Plugin example, it's only used for requests to denylisted servers). Several examples of setting up hooks are provided in Header-Based Plugin Examples and HTTP Transformations.
フィルタリング、ベーシック認証、リダイレクトを行うような ヘッダー操作プラグイン は通常 DNS ルックアップやリクエストヘッダの読込みの状態へのグローバルフックを持ちます。この先トランザクションに対して特定のアクションが実行される必要がある場合、プラグインは自身をトランザクションフックに追加します。 トランスフォームプラグイン は全トランザクションが、変換のために特別に使用されるトランザクションフックのタイプである トランスフォームフック により変換可能か確認するために、グローバルフックを必要とします。